Dev C++ Directx Tutorial
This time, Parallax Mapping will be discussed.
There's also OpenGL and DirectX tutorials. A good part of the resources are working with Dev-C Boboli. /dev-c-for-windows-10-reddit.html. Projectile.org is a site devoted to c/c, Unix, OpenGL and DirectX development. Their softwares are available with sources. A forum is also available for Q&A. The site is maintened by Zbalart which is a great friend of bloodshed.net. If you know DirectX, you can develop a DirectX app using native C and HLSL to take full advantage of graphics hardware. Waves trans x wide vst crack. Use this basic tutorial to get started with DirectX app development, then use the roadmap to continue exploring DirectX. Windows desktop app with C and DirectX. Mar 07, 2017 Visual Studio 2017 introduces a new “Game development with C” workload, making it easy to get tools you need for building high-quality games with C. Whether you’re using DirectX or powerful game engines such as Unreal Engine or Cocos2d, Visual Studio can install everything you need all at once to get you started quickly. DirectX 11 Tutorials Tutorial 1: Setting up DirectX 11 with Visual Studio. Tutorial 2: Creating a Framework and Window. Tutorial 3: Initializing DirectX 11. Tutorial 4: Buffers, Shaders, and HLSL. Tutorial 5: Texturing. Tutorial 6: Diffuse Lighting. Tutorial 7: 3D Model Rendering. Before we get started with this tutorial, you need to be familiar with these subjects. Microsoft C with Windows Runtime Language Extensions (C/CX). This is an update to Microsoft C that incorporates automatic reference counting, and is the language for developing a UWP games with DirectX 11.1 or later versions. This DirectX SDK release contains updates to tools, utilities, samples, documentation, and runtime debug files for x64 and x86 platforms. For additional information please see Microsoft DirectX Developer Center along with reviewing the Readme for last-minute updates. DirectX 11 Terrain Tutorials - Series 2 Tutorial 1: Grid and Camera Movement. Tutorial 6: Terrain Normal Mapping. Tutorial 7: Sky Domes. Tutorial 8: RAW Height Maps. Tutorial 9: Terrain Cells. Tutorial 10: Terrain Cell Culling. Tutorial 11: Height Based Movement. Tutorial 12: Terrain Mini-Maps. Tutorial 13: Procedural Terrain Texturing.
Moving from A to B, passing a static Object, will make the background appear to be moving |
Dev C++ Tutorial For Beginners
Directx 11 Tutorial
// we need to transform it to texture space. If you don't know what tbnMatrix
// is, read the tutorial over here.
/* VERTEX SHADER
outVS.toeyetangent = mul((camerapos - worldpos),tbnMatrix);
*/
// PIXEL SHADER
// The only thing we're doing here is skewing textures. We're only moving
// textures around. The higher a specific texel is, the more we move it.
// We'll be skewing in the direction of the view vector too.
// This is a texture coordinate offset. As I said it increases when height
// increases. Also required and worth mentioning is that we're moving along with
// the viewing direction, so multiply the offset by it.
// We also need to specify an effect multiplier. This normaly needs to about 0.4
float2 offset = toeyetangentin.xy*height*0.04f;
texcoordin += offset;
In its most basic form, this is all you need to do Parallax Mapping working. Let's sum things up, shall we?
- Textures lack depth. Depth is necessary to bring back a 3D feel to it.
- An important part of the depth illusion is Parallax. We want to bring it back into our textures.
- To do that, we need to obtain texel depth and the viewing direction. The viewing direction needs to be supplied in Tangent Space to the pixel shader.
- To do that we need to supply normals, binormals and tangents to the vertex shader. Combining these to a matrix and transposing it gives us the opportunity to transform from world to texture space.
- Then supply texture coordinates and the tangent space view vector to the pixel shader.
- Then sample depth from a regular heightmap. Multiply it by the view vector.
- And tada, you've got your texture coordinate offset. You're then supposed to use this texture coordinate for further sampling.
Now it does include a Parallax Map. It uses a multiplier of 0.4 and a single sample.
Yes, a single sample is all you need. No need to do PCF averaging or anything. Just a single tex instruction per pixel. But as you can see in the latter picture, there are some minor artifacts, especially on steeper viewing angles. To partially fix this, you need to include an offset constant, like this:
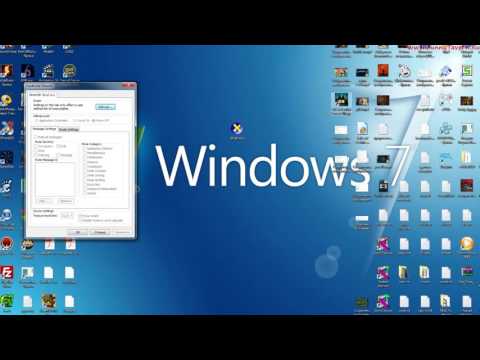
With this result:
Well, that's pretty much all there is to it. Have fun with it!
C++ Directx Example
- We need the surface orientation, because that's where the texture normals depend on.
- We know everything about our surface (a triangle).
- Any lighting component we need in PS (lightdir,viewdir,surfacedir) needs to be multiplied by the resulting matrix.
// Determine surface orientation by calculating triangles edges
Dev C++ Directx Tutorial 2017
Directx 11 Shader Tutorial
C++ Directx Tutorial
Dev C++ Directx Tutorials
// we're inside a Pixel Shader now
// texture coordinates are equal to the ones used for the diffuse color map
float3 normal = tex2D(normalmapsampler,coordin);
// color is stored in the [0,1] range (0 - 255), but we want our normals to be
// in the range op [-1,1].
// solution: multiply them by 2 (yields [0,2]) and substract one (yields [-1,1]).
normal = 2.0f*normal-1.0f;
// now that we've got our normal to work with, obtain (for example) lightdir
// for Phong shading
// lightdirtangentin is the same vector as lightdir in the VS around
// 20 lines above
float3 lightdir = normalize(lightdirtangentin);
/* use the variables as you would always do with your favourite lighting model */